Hello Everyone,
This is the part5 of my series on Maven, Spring and hibernate put together. This section is where we will actually feel some actions. this part 5 wasn’t planned from start, I introduced this step because it feels to me that there were so much to grab at once if I had published the original part5 (Only God knows how lengthy the original post could be). Instead of plumbing everything at once, I decided to split the plumbing per domain (Family, Person, Profile). So in this post, we will focus on the Family domain CRUD operations and some JSP section to show how to plumb together back end to front end.
- What is the use of service layer?
- Creating CRUD functionality for Familly through Services
- Creating FamilyController
- Creating List View needed by showList Action of the FamilyController
- Creating the form object for family
- Creating a success action and View
- Creating a new Family
- Processing the form
- Editing a family Details
- Deleting a family’s details
What is the use of service layer?
With the help of Spring-Data we have database operations covered. In our implementation that data layer is agnostic of whatever logic we want prior to any object persistence. Most of people have another Layer called Service Layer in which they put most of the Business Logic.
Creating CRUD functionality for Familly through Services
Let’s create FamilyService class in net.djomeda.tutorials.springdatamvcfamily.service package. This Service has regular Create-Read-Update-Delete (CRUD) methods and it should look like the snippet below:
[java]
//net.djomeda.tutorials.springdatamvcfamily.service.FamilyService.java
package net.djomeda.tutorials.springdatamvcfamily.service;
import java.util.List;
import java.util.UUID;
import net.djomeda.tutorials.springdatamvcfamily.model.Family;
import net.djomeda.tutorials.springdatamvcfamily.repository.FamilyRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.PageRequest;
import org.springframework.stereotype.Service;
/**
*
* @author kodjo-kuma Djomeda
*/
@Service
public class FamilyService {
@Autowired
FamilyRepository familyRepository;
/**
* Method to pull all records from the database
* @return List of Family Objects
*/
public List<Family> findAll(){
return familyRepository.findAll();
}
/**
* Method to pull records from the database page by page with the help of the number of records per page.
* @param pageSize total number of records per page.
* @param pageNumber number of page
* @return Page Object from Spring
*/
public Page findAll(int pageSize, int pageNumber){
return familyRepository.findAll(new PageRequest(pageNumber, pageSize));
}
/**
* Method to query by Id
* @param Id of the object to search
* @return queried Family Object
*/
public Family findById(String Id){
return familyRepository.findOne(Id);
}
/**
* Method to persist a family Object
* @param family object to persist
* @return saved Family object
*/
public Family save(Family family){
return familyRepository.save(family);
}
/**
* Method to persist a family Object by creating the Family Object before hand, taking parameters like name, tribe ,description
* @param name family name
* @param tribe family tribe
* @param description family description
* @return the newly persisted family object
*/
public Family create(String name, String tribe, String description){
Family newFamily = new Family();
newFamily.setID(UUID.randomUUID().toString());
newFamily.setName(name);
newFamily.setTribe(tribe);
newFamily.setDescription(description);
return familyRepository.save(newFamily);
}
/**
* Method to delete a particular object by its Id
* @param Id Family object to delete
*/
public void delete(String Id){
familyRepository.delete(Id);
}
/**
* Method to delete a particular object
* @param family object to delete
*/
public void delete(Family family){
familyRepository.delete(family);
}
}
[/java]
Creating FamilyController
Let’s head over the package net.djomeda.tutorials.springdatamvcfamily.controller for some actions (literally and figuratively). Let’s create FamilyController and let it look like the following:
[java]
//net.djomeda.tutorials.springdatamvcfaily.controller.FamilyController
package net.djomeda.tutorials.springdatamvcfamily.controller;
import javax.persistence.NoResultException;
import javax.validation.Valid;
import net.djomeda.tutorials.springdatamvcfamily.form.FamilyForm;
import net.djomeda.tutorials.springdatamvcfamily.model.Family;
import net.djomeda.tutorials.springdatamvcfamily.service.FamilyService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.dao.EmptyResultDataAccessException;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.servlet.ModelAndView;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
/**
*
* @author kodjo-kuma Djomeda
*/
@Controller
public class FamilyController {
@Autowired
FamilyService familyService;
@RequestMapping(value = {"/family","/family/list"})
public String showList(Model model){
model.addAttribute("families", familyService.findAll());
return "familyList";
}
}
[/java]
Creating List View needed by showList Action of the FamilyController
Right click on WEB-INF/pages/views folder choose new and choose JSP option form the context menu as shown in the pic below:
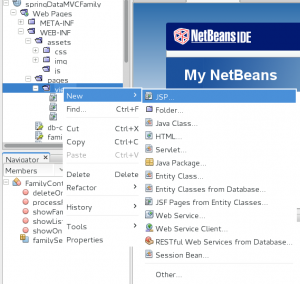
[html]
<%– springDataMVCFamily/src/main/webapp/WEB-INF/pages/views/familyList.jsp –%>
<%–
Document : familyList
Created on : Feb 16, 2014, 7:50:41 PM
Author : kodjo-kuma Djomeda
–%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@taglib uri="http://www.springframework.org/tags" prefix="spring" %>
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Welcome to family page</title>
</head>
<body>
<h1>Family List</h1>
<table border="1">
<tr>
<td>name</td>
<td>tribe</td>
<td>description</td>
<td colspan="3">Action</td>
</tr>
<c:forEach items="${families}" var="family">
<tr>
<td>${family.name}</td>
<td>${family.tribe}</td>
<td>${family.description}</td>
<td><a href="<spring:url value="/family/edit/${family.ID}" />">Edit</a></td>
<td><a href="<spring:url value="/family/delete/${family.ID}" />">Delete</a></td>
<td><a href="<spring:url value="#" />">show members</a></td>
</tr>
</c:forEach>
</table>
<br/>
<a href="<spring:url value="/"/>">Home</a> <a href="<spring:url value="/family/create"/>">Add</a>
</body>
</html>
[/html]
mapping showList action to its view
First of all, how does Spring know which view to render? remember when setting up the project we came to an arrangement with Spring where we instructed Spring with a particular preffix and suffix? see below
[xml]
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/pages/views/" />
<property name="suffix" value=".jsp" />
</bean>
[/xml]
With the settings above, we instrust Spring to look for the files in the folder “/WEB_INF/pages/views” and to append the “.jsp” to it. When you look closely, you would notice that “showList” action in FamilyController returns a name eg “familyList”. So Spring will go get “/WEB-INF/pages/views/familyList” + “.jsp”
When you build and run, it should look like the image below
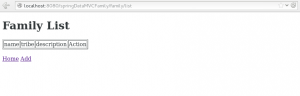
Creating the form object for family
In this section we are going to learn how to process a form, and especially from its creation to its validation, server side validation of course. Futher on this post, we will see how to manilpulate the form using spring taglib. Let’s head to the package net.djomeda.tutorials.springdatamvcfamily.form to create our FamilyForm object (POJO : Plain Old Java Object)
[java]
//net.djomeda.tutorials.springdatamvcfamily.form.FamilyForm.java
package net.djomeda.tutorials.springdatamvcfamily.form;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
/**
*
* @author kodjo-kuma Djomeda
*/
public class FamilyForm {
private String ID;
@NotEmpty(message = "Sorry Dude, Name can’t be empty")
@Size(min = 2, max= 20, message = "Sorry Bruv, Name length should be between 2 and 20 characters")
private String name;
@NotEmpty(message = "Tribe can’t be empty, naah mate, you can’t")
@Size(min = 2, max=20,message = "Common mhenn! Tribe length should be between 2 and 20 characters")
private String tribe;
private String description;
public FamilyForm(String name, String tribe, String description) {
this.name = name;
this.tribe = tribe;
this.description = description;
}
public FamilyForm(String ID, String name, String tribe, String description) {
this.ID = ID;
this.name = name;
this.tribe = tribe;
this.description = description;
}
public FamilyForm(String name, String tribe) {
this.name = name;
this.tribe = tribe;
}
public FamilyForm() {
}
/**
* @return the name
*/
public String getName() {
return name;
}
/**
* @param name the name to set
*/
public void setName(String name) {
this.name = name;
}
/**
* @return the tribe
*/
public String getTribe() {
return tribe;
}
/**
* @param tribe the tribe to set
*/
public void setTribe(String tribe) {
this.tribe = tribe;
}
/**
* @return the description
*/
public String getDescription() {
return description;
}
/**
* @param description the description to set
*/
public void setDescription(String description) {
this.description = description;
}
/**
* @return the ID
*/
public String getID() {
return ID;
}
/**
* @param ID the ID to set
*/
public void setID(String ID) {
this.ID = ID;
}
}
[/java]
Wait a minute! Everything was clair in this Form object (POJO) until wierd annotations shows up. Worry not! You are in safe hands. There were couple of @Size and @Max annotations in the form class. They are from the artifact hibernate-validator that we imported with maven into our project using the pom.xml file :
[xml]
<!–pom.xml–>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-validator</artifactId>
<version>4.0.2.GA</version>
</dependency>
[/xml]
The hibernate Validator is to help us set validation rules right on the class itself. Here we have used @NotEmpty to prevent submition of empty textbox. This together with spring will raise a flag when the submitted form doesn’t meet the validation rules and the form is usually sent back to the front end interface with the validation message when it’s configured properly eg : “Sorry Dude, Name can’t be empty”. In case you might want futher insight on Hibernate Validator, head to jboss documentation site for a little tour.
Creating a success action and View
In order to give some kind of feedback to the end users I have created a success View which takes advantage of RedirectAttribute that I will explain later. Create, Edit, and Delete of our CRUD make use of that action to display some meaningful answer.
showSuccess Action
[java]
//net.djomeda.tutorials.springdatamvcfaily.controller.FamilyController
//….
@RequestMapping(value = "/family/success")
public String showSuccess(Model model){
return "familySuccess";
}
[/java]
familySuccess View
[html]
<%–
Document : familySuccess
Created on : Feb 17, 2014, 1:10:35 PM
Author : joseph
–%>
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@taglib uri="http://www.springframework.org/tags" prefix="spring" %>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Family Creation Success</title>
</head>
<body>
<span>${message}</span>
<br/>
<a href="<spring:url value="/" />">Home</a> <a href="#" onclick="history.back()">Back</a>
</body>
</html>
[/html]
Creating a new Family
Making the form available to end users
In order to show the form and allow any end user to create a new record of family we need an action in the controller to send the form for a proper display. Add showFamilyCreateForm action to FamilyController as shown below:
[java]
//net.djomeda.tutorials.springdatamvcfaily.controller.FamilyController
//….
@RequestMapping(value = "/family/create")
public String showFamilyCreateForm(Model model){
FamilyForm form = new FamilyForm();
model.addAttribute("familyForm", form);
model.addAttribute("mgs","");
return "familyCreate";
}
//….
[/java]
In the action above, we have created an instance of FamilyForm object and assigned the key “familyForm” name to it when adding it to Spring org.springframework.ui.Model which would be responsible of making our form available to the view. In the view we will thus refer to the form by “familyForm”.
Creating familyCreate view for showFamilyCreateForm action of FamilyController
[html]
<%– springDataMVCFamily/src/main/webapp/WEB-INF/pages/views/familyCreate.jsp –%>
<%–
Document : familyCreate
Created on : Feb 17, 2014, 12:23:31 PM
Author : joseph
–%>
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<%@taglib uri="http://www.springframework.org/tags/form" prefix="form" %>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Create New Family Page</title>
</head>
<body>
<h1>Create New Family</h1>
<form:form action="processform" commandName="familyForm" class="form form-horizontal">
<c:if test="${not empty message}"></c:if>
<form:errors path="*" style="font-size:11px;color:red;" />
<table border="0">
<tr>
<td>Name:</td>
<td><form:input path="name" placeholder="Enter Family Name Here" /></td>
</tr>
<tr>
<td>Tribe:</td>
<td><form:input path="tribe" placeholder="Enter Tribe Name Here" /></td>
</tr>
<tr>
<td>Description :</td>
<td><form:textarea path="description" cols="25" rows="10"/> </td>
</tr>
<tr>
<td></td>
<td></td>
</tr>
<tr >
<td></td>
<td><button type="submit">submit</button><button type="reset">reset</button></td>
</tr>
</table>
</form:form>
</body>
</html>
[/html]
Validation of the Form
When you build and run an point the address to , it should look like the image below
Processing the form
In our form element there is ‘action=”processform”‘ which indicates what action/url should process the form. Explaining the whole mechanism here would make this post unecesarily long so I will try to explain brievly as much as I can. Let’s then define the processing action. In our FamilyController, add the following:
[java]
//net.djomeda.tutorials.springdatamvcfaily.controller.FamilyController
//…
@RequestMapping(value = "/family/processform")
public String processForm(@ModelAttribute("familyForm") @Valid FamilyForm form, BindingResult result,Model model, final RedirectAttributes redirectAttributes){
model.addAttribute("msg", "");
if(result.hasErrors()){
model.addAttribute("familyForm", form);
return "familyCreate";
}
try{
familyService.create(form.getName(),form.getTribe(), form.getDescription());
redirectAttributes.addFlashAttribute("message", "Created Successfully");
}catch(Exception ex){
model.addAttribute("msg", "Hi! an error Occured, disturb your chakra to wake up from genjutsu");
model.addAttribute("familyForm", form);
return "familyCreate";
}
return "redirect:/family/success";
}
// …
[/java]
There are couple of things here that need some attention. Those are @Valid, @ModelAttribute, BindingResult, RedirectAttributes
- @Valid isn’t a Spring annotation but a JSR-303 annotation. So simply it’s for validation purpose (of our FamilyForm object)
- @ModelAttribute refers to a property of the Model object (the M in MVC we used familyForm attribute to pass the form instance of our FamilyForm object)
- BindingResult is used for error registration, I mean by that any validation error. Together with ModelAttribute allows the display of the error of the field whose validation failed.
- RedirectAttributes It’s use to register the message for the next redirected url.
Again you can read more on these on your own later.
Let’s try to create a Family Record without Tribe field
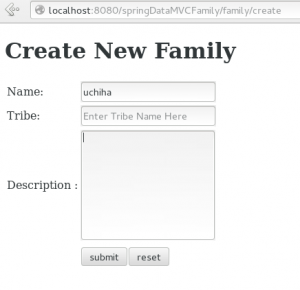
After Clicking on submit button, the image below is what shows on the page after Validation:
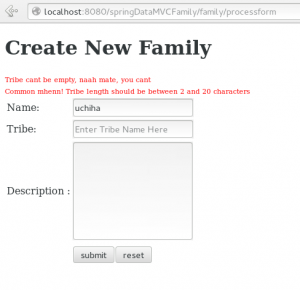
Let’s Create the record again with correct with all requirements.
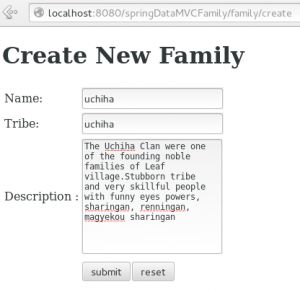
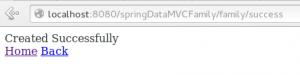

Editing a family Details
After adding a couple of other families, Let’s decide on which one to edit. Here again we need to show the form before processing it. So we will need 2 actions to complete this steps.
Displaying the form to edit
In a number of actions in the FamilyController I only returned the name of the view file and used org.springframework.ui.Model to send data to the view. But for EditOne action that we will create below, I deliberately used another technique which is ModelAndView. That is me showing off totally … well a little. Fact is I wanted to show how things could be done differently and for there is no actually preference in returning a view name or a ModelAndView instance. See below the snippet of the action.
[java]
//net.djomeda.tutorials.springdatamvcfaily.controller.FamilyController
// …
@RequestMapping(value = "/family/edit/{familyId}")
public ModelAndView editOne(@PathVariable("familyId") String familyId){
ModelAndView mv = new ModelAndView("familyEdit");
try{
Family family = familyService.findById(familyId);
FamilyForm form = new FamilyForm(family.getID(),family.getName(), family.getTribe(), family.getDescription());
mv.addObject("form", form);
}catch(NoResultException nre){
mv.addObject("msg", "The family you wanted so bad to edit is no more");
}
return mv;
}
//…
[/java]
Here we needed to pass to the edit method the ID of the item we want to edit. We have chosen to include the id in the url (REST way or cute url) as opposed to have a query string “/family/edit?id=blablabla”. For that to happen we use spring annotation @PathValiable and together with spring helpers that id becomes the argument of our action method. We went to fetch for the actual record and created a form out of it and then sent it to the View through ModelAndView, but it could be via the simpler Model object we have been using so far.
some would have avoided the step of transferring the control to a different object FamilyForm for example and just work with the Family object/Model directly from the form
[java]
//…
@RequestMapping(value = "/family/edit/{familyId}")
public ModelAndView editOne(@PathVariable("familyId") String familyId)
// …
[/java]
Processing Edit form
Notice that this time as well we used “form” as Model attribute so our annotation of @ModelAttribute would be “form” as opposed to “familyForm” it’s just a matter of choice.
before is the code for processing the editing of the form
[java]
//net.djomeda.tutorials.springdatamvcfaily.controller.FamilyController
// …
@RequestMapping(value = "/family/processeditform", method = RequestMethod.POST)
public String processEditForm(@ModelAttribute("form") @Valid FamilyForm form, BindingResult result,Model model, final RedirectAttributes redirectAttributes){
model.addAttribute("msg", "");
if(result.hasErrors()){
model.addAttribute("form", form);
return "familyEdit";
}
try{
Family edited = new Family();
edited.setID(form.getID());
edited.setName(form.getName());
edited.setTribe(form.getTribe());
edited.setDescription(form.getDescription());
familyService.save(edited);
redirectAttributes.addFlashAttribute("message", "Edited Successfully");
}catch(Exception ex){
model.addAttribute("msg", "Hi! an error Occured, disturb your chakra to wake up from genjutsu ");
model.addAttribute("form", form);
return "familyEdit";
}
return "redirect:/family/success";
}
//…
[/java]
Creating familyEdit view for the edition
[html]
<%– springDataMVCFamily/src/main/webapp/WEB-INF/pages/views/familyEdit.jsp –%>
<%–
Document : familyEdit
Created on : Feb 17, 2014, 12:23:31 PM
Author : joseph
–%>
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<%@taglib uri="http://www.springframework.org/tags/form" prefix="form" %>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Edit Family Page</title>
</head>
<body>
<h1>Edit ${form.name} Family</h1>
<form:form action="/family/processeditform" commandName="form" method="POST" class="form form-horizontal">
<%–<c:if test="${not empty message}"></c:if>–%>
<span style="font-size:11px;color:red;">${msg}</span>
<form:errors path="*" style="font-size:11px;color:red;" />
<table border="0">
<tr>
<td>Name:</td>
<td><form:input path="name" placeholder="Enter Family Name Here" /></td>
</tr>
<tr>
<td>Tribe:</td>
<td><form:input path="tribe" placeholder="Enter Tribe Name Here" /></td>
</tr>
<tr>
<td>Description :</td>
<td><form:textarea path="description" cols="25" rows="10"/> </td>
</tr>
<tr>
<td><form:hidden path="ID" /></td>
<td></td>
</tr>
<tr >
<td></td>
<td><button type="submit">edit</button><button type="reset">reset</button></td>
</tr>
</table>
</form:form>
</body>
</html>
[/html]
Let’s click on the edit link of one of the items of our family list page . In my example I will use aburame’s clan/tribe.
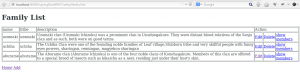
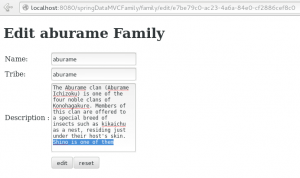
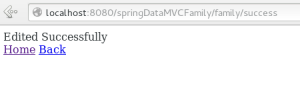
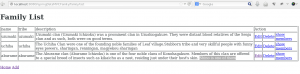
Deleting a family’s details
Deleting an object is a little similar to the edit part in the sense that you need to point out what exactly what to edit or to delete. We have actually in the familyList.jsp included the link to those actions so that it reflect the rule we have set with @MappingRequest for a particular action.
for example on the deleteOne action of the FamilyController the request mapping is “/family/delete/{familyId}” . Since in the list we are showing all families, we have links that would help perform some actions on them. So we had Edit, Delete and Show members list (disable for now though). We have accomplished that with the snippet of the familyList.jsp(We already had this) code below :
[html]
<td><a href="<spring:url value="/family/delete/${family.ID}" />">Delete</a></td>
[/html]
Creating deleteOne action
Below is the deleteOne action codes :
[java]
//net.djomeda.tutorials.springdatamvcfaily.controller.FamilyController
// …
@RequestMapping(value = "/family/delete/{familyId}")
public String deleteOne(@PathVariable("familyId") String familyId, Model model){
try{
familyService.delete(familyId);
model.addAttribute("msg", "jay!, we destroyed the family");
} catch(EmptyResultDataAccessException erdae){
model.addAttribute("msg", "ok nephew!, the data you are trying to delete does not exist");
} catch(Exception e){
model.addAttribute("msg","Sorry Mate, there seems to be an issue.");
}
return "familyDelete";
}
//…
[/java]
Here we tried deleting the family by its ID assuming the object of that ID is actually there which could be wrong BUT we put the code in a try and catch block then we caught the exception of it not being there. Check the EmptyResultDataAccessException catch block.
The full controller looks like below
[java]
//net.djomeda.tutorials.springdatamvcfaily.controller.FamilyController
package net.djomeda.tutorials.springdatamvcfamily.controller;
import javax.persistence.NoResultException;
import javax.validation.Valid;
import net.djomeda.tutorials.springdatamvcfamily.form.FamilyForm;
import net.djomeda.tutorials.springdatamvcfamily.model.Family;
import net.djomeda.tutorials.springdatamvcfamily.service.FamilyService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.dao.EmptyResultDataAccessException;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.servlet.ModelAndView;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
/**
*
* @author joseph
*/
@Controller
public class FamilyController {
@Autowired
FamilyService familyService;
@RequestMapping(value = {"/family","/family/list"})
public String showList(Model model){
model.addAttribute("families", familyService.findAll());
return "familyList";
}
@RequestMapping(value = "/family/show/{familyId}")
public String showOne(@PathVariable("familyId") String familyId, Model model){
model.addAttribute("family", familyService.findById(familyId));
return "familyOne";
}
@RequestMapping(value = "/family/delete/{familyId}")
public String deleteOne(@PathVariable("familyId") String familyId, Model model){
try{
familyService.delete(familyId);
model.addAttribute("msg", "jay!, we destroyed the family");
} catch(EmptyResultDataAccessException erdae){
model.addAttribute("msg", "ok nephew!, the data you are trying to delete does not exist");
} catch(Exception e){
model.addAttribute("msg","Sorry Mate, there seems to be an issue.");
}
return "familyDelete";
}
@RequestMapping(value = "/family/edit/{familyId}")
public ModelAndView editOne(@PathVariable("familyId") String familyId){
ModelAndView mv = new ModelAndView("familyEdit");
try{
Family family = familyService.findById(familyId);
FamilyForm form = new FamilyForm(family.getID(),family.getName(), family.getTribe(), family.getDescription());
mv.addObject("form", form);
}catch(NoResultException nre){
mv.addObject("msg", "The family you wanted so bad to edit is no more");
}
return mv;
}
@RequestMapping(value = "/family/processeditform", method = RequestMethod.POST)
public String processEditForm(@ModelAttribute("form") @Valid FamilyForm form, BindingResult result,Model model, final RedirectAttributes redirectAttributes){
model.addAttribute("msg", "");
if(result.hasErrors()){
model.addAttribute("form", form);
return "familyEdit";
}
try{
Family edited = new Family();
edited.setID(form.getID());
edited.setName(form.getName());
edited.setTribe(form.getTribe());
edited.setDescription(form.getDescription());
familyService.save(edited);
redirectAttributes.addFlashAttribute("message", "Edited Successfully");
}catch(Exception ex){
model.addAttribute("msg", "Hi! an error Occured, disturb your chakra to wake up from genjutsu ");
model.addAttribute("form", form);
return "familyEdit";
}
return "redirect:/family/success";
}
@RequestMapping(value = "/family/create")
public String showFamilyCreateForm(Model model){
FamilyForm form = new FamilyForm();
model.addAttribute("familyForm", form);
model.addAttribute("mgs","");
return "familyCreate";
}
@RequestMapping(value = "/family/processform")
public String processForm(@ModelAttribute("familyForm") @Valid FamilyForm form, BindingResult result,Model model, final RedirectAttributes redirectAttributes){
model.addAttribute("msg", "");
if(result.hasErrors()){
model.addAttribute("familyForm", form);
return "familyCreate";
}
try{
familyService.create(form.getName(),form.getTribe(), form.getDescription());
redirectAttributes.addFlashAttribute("message", "Created Successfully");
}catch(Exception ex){
model.addAttribute("msg", "Hi! an error Occured, disturb your chakra to wake up from genjutsu");
model.addAttribute("familyForm", form);
return "familyCreate";
}
return "redirect:/family/success";
}
@RequestMapping(value = "/family/success")
public String showSuccess(Model model){
return "familySuccess";
}
}
[/java]
So that’s all folks, I hope this is not too long and boring. This is real practice time so I hope you enjoyed it ^_^ .
You can find the files on my bitbucket . Click here to download